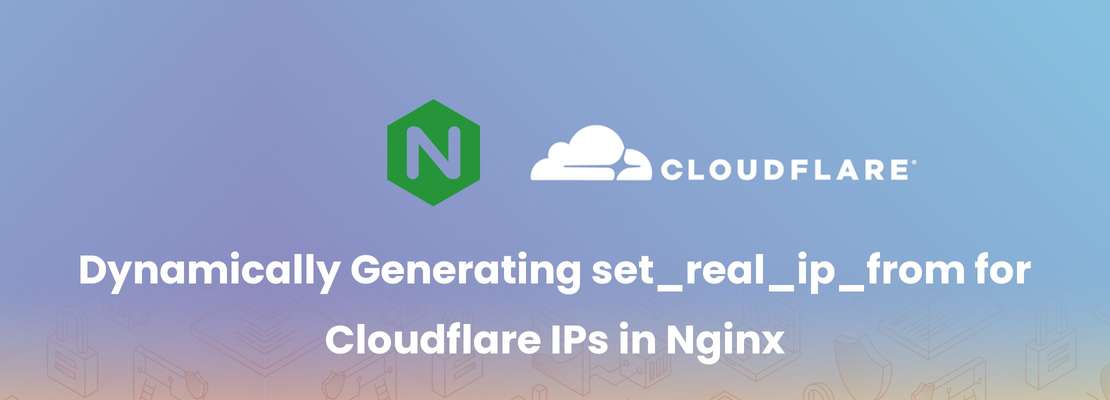
- By: Max Mastalerz
- January 14, 2024
Dynamically Generating set_real_ip_from for Cloudflare IPs in Nginx
When your website traffic is directed through Cloudflare's DNS proxy, Nginx will not provide you with very useful information on the original visitor. Instead you will see an IP address coming from Cloudflare. Luckily there is a solution to this.
If you are NOT using nginx's docker image, you will need to build nginx with the following configuration parameter: --with-http_realip_module . If you are using the nginx
docker image, the module is already available to you. Once the module is available to you, you can use it in your nginx.conf
file. For Docker, take the currently generated nginx.conf found on your nginx container and update it like so (See area with comments):
user nginx;
worker_processes auto;
error_log /var/log/nginx/error.log notice;
pid /var/run/nginx.pid;
events {
worker_connections 1024;
}
http {
include /etc/nginx/mime.types;
default_type application/octet-stream;
log_format main '$remote_addr - $remote_user [$time_local] "$request" '
'$status $body_bytes_sent "$http_referer" '
'"$http_user_agent" "$http_x_forwarded_for" "$http_cf_connecting_ip"';
access_log /var/log/nginx/access.log main;
sendfile on;
#tcp_nopush on;
keepalive_timeout 65;
#gzip on;
# Real IP Module Configuration. Cloudflare IPs listed at https://www.cloudflare.com/ips-v4/# and https://www.cloudflare.com/ips-v6/#
# custom-nginx.sh updates these on start and every 6 hours.
set_real_ip_from dummy_ip_will_be_replaced_dynamically;
set_real_ip_from dummy_ip_will_be_replaced_dynamically;
real_ip_header CF-Connecting-IP; #Note: this line is also replaced dynamically.
include /etc/nginx/conf.d/*.conf;
}
In your Dockerfile we can overwrite the default nginx.conf with:
COPY ./<your_local_project_folder>/nginx.conf /etc/nginx/nginx.conf
Now, we just need to dynamically replace the set_real_ip_from dummy_ip_will_be_replaced_dynamically;
lines with CloudFlare's ip blocks.
Let's make our own custom-nginx.sh
start script:
#!/bin/sh
update_cloudflare_ips() {
# Fetch the latest Cloudflare IP addresses (replace 'curl' with 'wget' if needed)
cloudflare_ips_v4=$(curl -s https://www.cloudflare.com/ips-v4)
cloudflare_ips_v6=$(curl -s https://www.cloudflare.com/ips-v6)
# Create a string with set_real_ip_from lines for each IP address
set_real_ip_lines=""
for ip in $cloudflare_ips_v4 $cloudflare_ips_v6; do
set_real_ip_lines="${set_real_ip_lines}set_real_ip_from ${ip};\n "
done
# Update nginx.conf with the new set_real_ip_from values
sed -i '/set_real_ip_from/d' /etc/nginx/nginx.conf
sed -i "/real_ip_header/c\ ${set_real_ip_lines}real_ip_header CF-Connecting-IP;" /etc/nginx/nginx.conf
}
reload_nginx() {
while true; do
sleep 6h
update_cloudflare_ips
echo "Reloading Nginx..."
nginx -s reload
echo "Nginx reloaded."
done
}
update_cloudflare_ips
# Start Nginx
echo "Starting Nginx..."
nginx -g "daemon off;" &
NGINX_PID=$!
# Run the reload function in the background
reload_nginx &
# Wait for the main Nginx process to finish
wait $NGINX_PID
And now we can use that to run nginx instead. Here is our updated Dockerfile
:
FROM nginx:stable-alpine
COPY ./<your_local_project_folder>/nginx.conf /etc/nginx/nginx.conf
...
COPY ./<your_local_project_folder>/custom-nginx.sh /usr/sbin
RUN chmod +x /usr/sbin/custom-nginx.sh
ENTRYPOINT ["/usr/sbin/custom-nginx.sh"]
If you found this post helpful let me know!